How to Create Controller in Magento 2
Controller specially is one of the important thing in Module development series, and PHP MVC Framework in general. It functionarity is that received request, process and render page.
In Magento 2 Controller has one or more files in Controller folder of module, it includes actions of class which contain execute()
method. There are 2 different controllers, they are frontend controller and backend controller. They are generally similar of workflow, but admin controller is a little different. There is a checking permission method in admin controller, it calls form key
.
How controller work?
It receive an request from end-user (browser or comamnd line), for example:
http://example.com/route_name/controller/action
route_name
is a unique name which is set in routes.xml.controller
is the folder inside Controller folder.action
is a class with execute method to process request.
One of the important in Magento system is frontController (Magento\Framework\App\FrontController
), it alway receives request then route controller, action by route_name
Let’s take an example of routing an request:
foreach ($this->_routerList as $router) {
try {
$actionInstance = $router->match($request);
…
}
If there is an action of controller class found, execute()
method will be run.
How to create a controller in Magento 2?
To Create Controller in Magento 2:
- Step 1: Create routes.xml file
- Step 2: Create controller file
- Step 3: Create controller Layout file
- Step 4: Create controller Block file
- Step 5: Create controller template file
- Step 6: Flush Magento cache
- Step 7: Run a test new controller
To create a controller, we need to create a folder inside Controller
folder of module and declare an action class inside it. For example, we create a index controller
and a index
action for module Mageplaza_HelloWorld
:
Step 1: Create routes.xml file.
File: app/code/Mageplaza/HelloWorld/etc/frontend/routes.xml
<?xml version="1.0" ?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:App/etc/routes.xsd">
<router id="standard">
<route frontName="helloworld" id="helloworld">
<module name="Mageplaza_HelloWorld"/>
</route>
</router>
</config>
In the previous How to Create Module in Magento 2 , we created file routes.xml. If you created it, you can ignore this step.
Step 2: Create controller file
File: app/code/Mageplaza/HelloWorld/Controller/Index/Index.php
<?php
namespace Mageplaza\HelloWorld\Controller\Index;
class Index extends \Magento\Framework\App\Action\Action
{
protected $_pageFactory;
public function __construct(
\Magento\Framework\App\Action\Context $context,
\Magento\Framework\View\Result\PageFactory $pageFactory)
{
$this->_pageFactory = $pageFactory;
return parent::__construct($context);
}
public function execute()
{
return $this->_pageFactory->create();
}
}
As you see, all controllers must be extended \Magento\Framework\App\Action\Action
class which has dispatch method which will call execute()
method in action class. In this execute()
method, we will write all of our controller logic and will return response for the request.
Step 3: Create Layout file
File: app/code/Mageplaza/HelloWorld/view/frontend/layout/helloworld_index_index.xml
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" layout="1column" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<referenceContainer name="content">
<block class="Mageplaza\HelloWorld\Block\Index" name="helloworld_index_index" template="Mageplaza_HelloWorld::index.phtml" />
</referenceContainer>
</page>
Step 4: Create Block file
File: app/code/Mageplaza/HelloWorld/Block/Index.php
<?php
namespace Mageplaza\HelloWorld\Block;
class Index extends \Magento\Framework\View\Element\Template
{
}
Step 5: Create template file
File: app/code/Mageplaza/HelloWorld/view/frontend/templates/index.phtml
<h2>Welcome to Mageplaza.com</h2>
We can learn more View: Layout, Block, Template in this topic.
Step 6: Flush Magento cache
How to flush Magento cache here
Step 7: Run a test
Let’s open browser and navigate to
http://<yourhost.com>/helloworld/index/index
or
http://<yourhost.com>/helloworld/
Permission - ACL
There is a checking permission method in admin controller. Let’s take an example:
protected function _isAllowed()
{
return $this->_authorization->isAllowed('Magento_AdminNotification::show_list');
}
It will check the current user has right to access this action or not, learn more Admin ACL Access Control Lists
Other methods in Magento 2 Controller
_forward() and _redirect() action.
\Magento\Framework\App\Action\Action
class provide us 2 important methods: _forward
and _redirect
.
Forward method
_forward()
protected function will edit the request to transfer it to another controller/action
class. This will not change the request url. For example, we have 2 actions Forward and Hello World like this:
namespace Mageplaza\HelloWorld\Controller\Test;
class Forward extends \Magento\Framework\App\Action\Action
{
public function execute()
{
$this->_forward('hello');
}
}
If you make a request to http://example.com/route_name/test/forward
, here are result will be displied on the screen.
Hello World! Welcome to Mageplaza.com
You can also change the controller, module and set param for the request when forward. Please check the _forward()
function for more information:
protected function _forward($action, $controller = null, $module = null, array $params = null)
{
$request = $this->getRequest();
$request->initForward();
if (isset($params)) {
$request->setParams($params);
}
if (isset($controller)) {
$request->setControllerName($controller);
// Module should only be reset if controller has been specified
if (isset($module)) {
$request->setModuleName($module);
}
}
$request->setActionName($action);
$request->setDispatched(false);
}
Redirect method
This method will transfer to another controller/action
class and also change the response header and the request url. With above example, if we replace _forward()
method by this _redirect()
method:
$this->_redirect('*/*/hello');
Then after access from the url http://example.com/route_name/test/forward
, the url will be change to http://example.com/route_name/test/hello
and show the message Hello World! Welcome to Mageplaza.com
on the screen.
If you got this error message: Exception printing is disabled by default for security reasons, this topic may help.
Now it comes to the end of Create a controller
topic, in the next tutorial, I will show you how to create a model in Magento 2.
Related Post
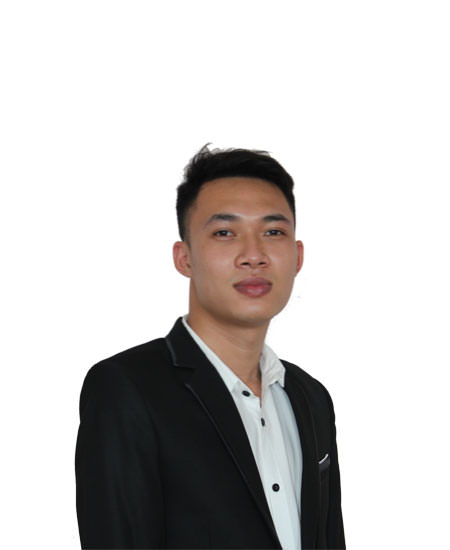
- How to create a simple Hello World module for Magento 2
- Magento 2 Block Template Ultimate Guides
- How to Create Module in Magento 2
- How to create CRUD Models in Magento 2
- How to Create Magento 2 Block, Layout and Templates
- Configuration - System.xml
- How To Create Admin Menu In Magento 2
- Admin ACL
- Admin Grid
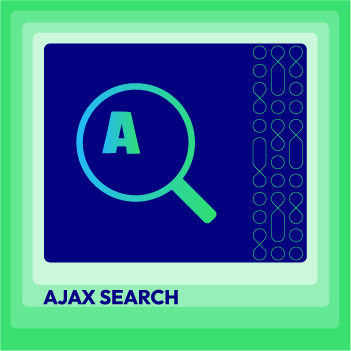
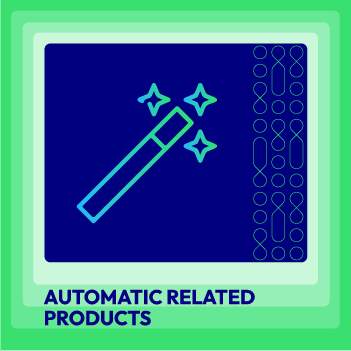
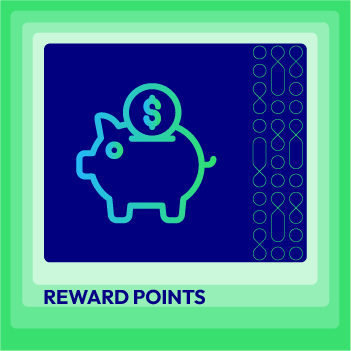
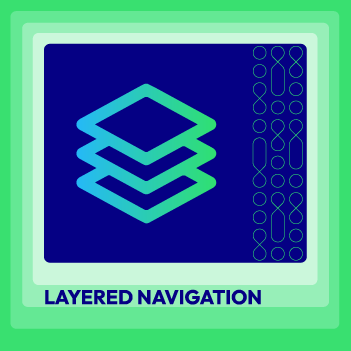
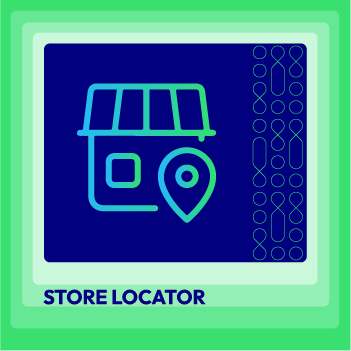
People also searched for
- magento 2 controller
- controller for magento 2
- how to create controller in Magento 2
- magento 2 create controller
- Custom Controllers module
- admin controller
- frontend controller
- Controller action
- Difference between admin and front controller
- How to create custom controller
- Admin and Front controllers
- create controller magento 2
- magento 2 custom controller
- create controller in magento 2
- magento2 controller
- magento 2 add controller
- magento 2 frontend controller
- magento 2 create frontend controller
- magento 2 controller url
- magento 2 create admin controller
- magento 2 admin controller
- magento 2 create ajax controller
- magento 2 call controller action
- magento 2 rewrite controller
- magento 2 call controller action from phtml
- magento 2 create controller action
- 2.3.x, 2.4.x