How to Get size of product image in Magento 2
This topic demonstrates how to get size of product image in Magento 2.
Getting the size of product image is the next topic you also need to know in Magento 2.
Actually, product image size is a good attribute to your site overall performance. It makes your products closer to customers with a real visualization of your products with details. Having beautiful and professional products images helps you easily attracts customers and stand out among different websies.
To show you clearly, I highly recommend the following example which mention how to display as well as get the size (height and width) of the uploaded product.
2 Steps to retrieve the size of product image
Now follow the instruction below to successfully resize a product image in Magento 2.
Step 1: Upload the product
Open your custom module (Mageplaza_HelloWorld
), insert object of \Magento\Catalog\Model\ProductRepository
class in the constructor of the module’s block class
app/code/Mageplaza/HelloWorld/Block/HelloWorld.php
<?php
namespace Mageplaza\HelloWorld\Block;
class HelloWorld extends \Magento\Framework\View\Element\Template
{
protected $_productRepository;
protected $_productImageHelper;
public function __construct(
\Magento\Backend\Block\Template\Context $context,
\Magento\Catalog\Model\ProductRepository $productRepository,
\Magento\Catalog\Helper\Image $productImageHelper,
array $data = []
)
{
$this->_productRepository = $productRepository;
$this->_productImageHelper= $productImageHelper;
parent::__construct($context, $data);
}
public function getProductById($id)
{
return $this->_productRepository->getById($id);
}
public function getProductBySku($sku)
{
return $this->_productRepository->get($sku);
}
/**
* Retrieve image width
*
* @return int|null
*/
public function getImageOriginalWidth($product, $imageId, $attributes = [])
{
return $this->_productImageHelper->init($product, $imageId, $attributes)->getWidth();
}
/**
* Retrieve image height
*
* @return int|null
*/
public function getImageOriginalHeight($product, $imageId, $attributes = [])
{
return $this->_productImageHelper->init($product, $imageId, $attributes)->getHeight();
}
}
?>
Step 2: Retrieve the size of the product image
It is possible to get and print the height and width of product_small_image
, product_base_image
and product_thumbnail_image
, etc. Please run the below code:
$id = YOUR_PRODUCT_ID;
$sku = 'YOUR_PRODUCT_SKU';
$_product = $block->getProductById($id);
$_product = $block->getProductBySku($sku);
echo $block->getImageOriginalWidth($_product, 'product_small_image') . '<br />';
echo $block->getImageOriginalHeight($_product, 'product_small_image') . '<br />';
With LUMA as the default frontend template, you need to open the layout YOUR_MAGENTO_ROOT/vendor/magento/theme-frontend-luma/etc/view.xml
, an you can change the height and width for different media images.
<media>
<images module="Magento_Catalog">
<image id="bundled_product_customization_page" type="thumbnail">
<width>140</width>
<height>140</height>
</image>
<image id="cart_cross_sell_products" type="thumbnail">
<width>200</width>
<height>248</height>
</image>
<image id="cart_page_product_thumbnail" type="small_image">
<width>165</width>
<height>165</height>
</image>
<image id="category_page_grid" type="small_image">
<width>240</width>
<height>300</height>
</image>
<image id="category_page_grid-1" type="small_image">
<width>240</width>
<height>300</height>
</image>
<image id="category_page_list" type="small_image">
<width>240</width>
<height>300</height>
</image>
<image id="customer_account_my_tags_tag_view" type="small_image">
<width>100</width>
<height>100</height>
</image>
<image id="customer_account_product_review_page" type="image">
<width>285</width>
<height>285</height>
</image>
<image id="customer_shared_wishlist" type="small_image">
<width>113</width>
<height>113</height>
</image>
<image id="gift_messages_checkout_small_image" type="small_image">
<width>75</width>
<height>75</height>
</image>
<image id="gift_messages_checkout_thumbnail" type="thumbnail">
<width>100</width>
<height>100</height>
</image>
<image id="mini_cart_product_thumbnail" type="thumbnail">
<width>75</width>
<height>75</height>
</image>
<image id="new_products_content_widget_grid" type="small_image">
<width>240</width>
<height>300</height>
</image>
<image id="new_products_content_widget_list" type="small_image">
<width>270</width>
<height>340</height>
</image>
<image id="new_products_images_only_widget" type="small_image">
<width>78</width>
<height>78</height>
</image>
<image id="product_base_image" type="image">
<width>265</width>
<height>265</height>
</image>
<image id="product_comparison_list" type="small_image">
<width>140</width>
<height>140</height>
</image>
<image id="product_page_image_large" type="image"/>
<image id="product_page_image_medium" type="image">
<width>700</width>
<height>560</height>
</image>
<image id="product_page_image_small" type="thumbnail">
<width>88</width>
<height>110</height>
</image>
<image id="product_page_main_image" type="image">
<width>700</width>
<height>560</height>
</image>
<image id="product_page_main_image_default" type="image">
<width>700</width>
<height>560</height>
</image>
<image id="product_page_more_views" type="thumbnail">
<width>88</width>
<height>110</height>
</image>
<image id="product_stock_alert_email_product_image" type="small_image">
<width>76</width>
<height>76</height>
</image>
<image id="product_small_image" type="small_image">
<width>135</width>
<height>135</height>
</image>
<image id="product_thumbnail_image" type="thumbnail">
<width>75</width>
<height>75</height>
</image>
<image id="recently_compared_products_grid_content_widget" type="small_image">
<width>240</width>
<height>300</height>
</image>
<image id="recently_compared_products_images_names_widget" type="thumbnail">
<width>75</width>
<height>90</height>
</image>
<image id="recently_compared_products_images_only_widget" type="thumbnail">
<width>76</width>
<height>76</height>
</image>
<image id="recently_compared_products_list_content_widget" type="small_image">
<width>270</width>
<height>340</height>
</image>
<image id="recently_viewed_products_grid_content_widget" type="small_image">
<width>240</width>
<height>300</height>
</image>
<image id="recently_viewed_products_images_names_widget" type="small_image">
<width>75</width>
<height>90</height>
</image>
<image id="recently_viewed_products_images_only_widget" type="small_image">
<width>76</width>
<height>76</height>
</image>
<image id="recently_viewed_products_list_content_widget" type="small_image">
<width>270</width>
<height>340</height>
</image>
<image id="related_products_list" type="small_image">
<width>152</width>
<height>190</height>
</image>
<image id="review_page_product_image" type="small_image">
<width>285</width>
<height>285</height>
</image>
<image id="rss_thumbnail" type="thumbnail">
<width>75</width>
<height>75</height>
</image>
<image id="sendfriend_small_image" type="small_image">
<width>75</width>
<height>75</height>
</image>
<image id="shared_wishlist_email" type="small_image">
<width>135</width>
<height>135</height>
</image>
<image id="side_column_widget_product_thumbnail" type="thumbnail">
<width>75</width>
<height>90</height>
</image>
<image id="upsell_products_list" type="small_image">
<width>152</width>
<height>190</height>
</image>
<image id="wishlist_sidebar_block" type="thumbnail">
<width>75</width>
<height>90</height>
</image>
<image id="wishlist_small_image" type="small_image">
<width>113</width>
<height>113</height>
</image>
<image id="wishlist_thumbnail" type="small_image">
<width>240</width>
<height>300</height>
</image>
</images>
</media>
Wrap up
That’s all about changing products’ image sizes in Magento 2. I hope you successfully resize your product images following this tutorial. If you have any questions or want to discuss more about this topic, feel free to let me know.
Related Topics
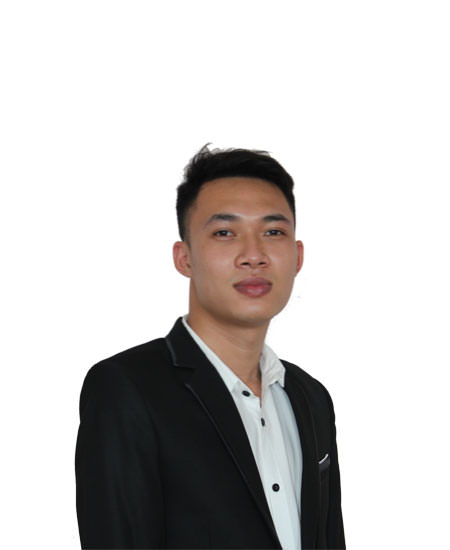
- How to create a simple Hello World module for Magento 2
- Magento 2 Block Template Ultimate Guides
- How to Create Module in Magento 2
- How to Create Controller in Magento 2
- How to create CRUD Models in Magento 2
- How to Create Magento 2 Block, Layout and Templates
- Configuration - System.xml
- How To Create Admin Menu In Magento 2
- Admin ACL
- Admin Grid
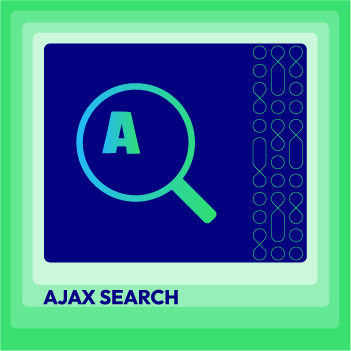
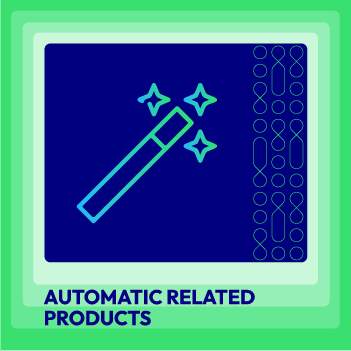
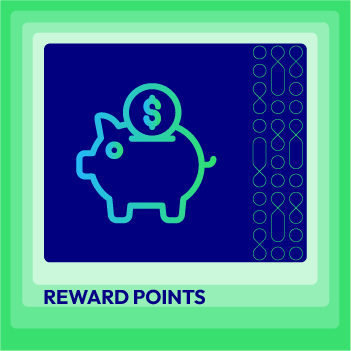
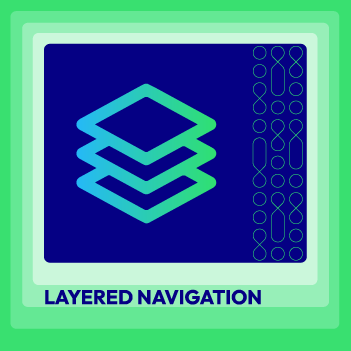
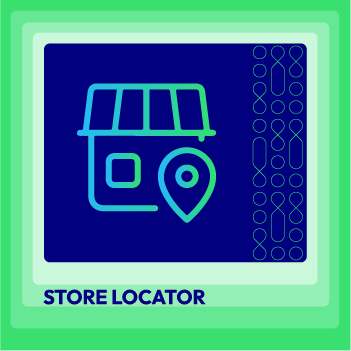
People also searched for
- magento 2 get product image in phtml
- magento 2 get product base image
- get product image magento 2
- get product image in magento 2
- magento 2 product image size
- get image product magento 2
- magento 2 get product thumbnail image
- magento 2 get product thumbnail image url in phtml
- magento 2 get product media gallery images
- magento 2 product image
- magento 2 rest api get product image
- get product image url in magento 2
- magento get product image
- magento 2 get product image url from collection
- how to get product image in magento 2
- magento 2 thumbnail image size
- magento 2 get product thumbnail image url
- magento 2 image helper
- magento 2 get product gallery images
- magento 2 get thumbnail image url
- magento 2 get thumbnail image
- magento 2 get product images
- magento 2 default product image size
- magento 2 gallery image size
- magento 2 get all product images
- 2.3.x, 2.4.x