How to Get Order Collections with Ffilter in Magento 2
Vinh Jacker | 03-17-2025
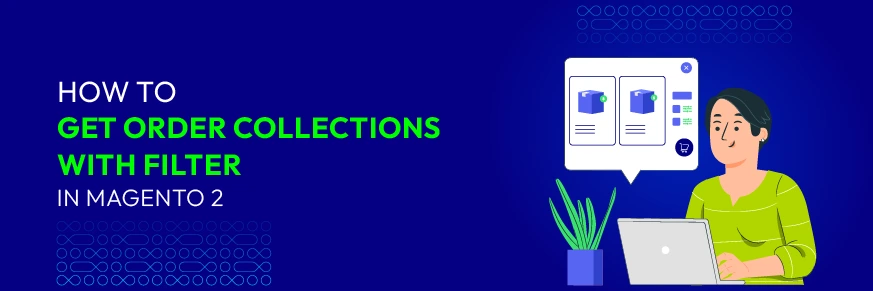
Managing orders efficiently is crucial for Magento 2 store owners. Filtering orders by attributes like customer, date, status, and payment method can help streamline business processes and improve analysis. In this guide, we’ll walk you through multiple methods to fetch filtered order collections in Magento 2, complete with practical examples and insights.
Read more about Get Product Collection Filter by Visibility
Method 1: Get All Order Collection with filters
To get all order collections with filters, start by creating the necessary class in your custom module. Ggo to the following path Mageplaza/HelloWorld/Block/Orders.php
and create an Orders.php
file.
<?php
namespace Mageplaza\HelloWorld\Block;
class Products extends \Magento\Framework\View\Element\Template
{
protected $_orderCollectionFactory;
public function __construct(
Magento\Framework\App\Action\Context $context,
\Magento\Sales\Model\ResourceModel\Order\CollectionFactory $orderCollectionFactory
) {
$this->_orderCollectionFactory = $orderCollectionFactory;
parent::__construct($context);
}
public function getOrderCollection()
{
$collection = $this->_orderCollectionFactory->create()
->addAttributeToSelect('*')
->addFieldToFilter($field, $condition); //Add condition if you wish
return $collection;
}
public function getOrderCollectionByCustomerId($customerId)
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('status',
['in' => $this->_orderConfig->getVisibleOnFrontStatuses()]
)
->setOrder(
'created_at',
'desc'
);
return $collection;
}
}
Method 2: Get All Order Collection Filter by Customer
While developing Magento 2 extensions at the frontend like My Account, you might need to display existing customer orders in a backend grid. Here is how we can get all orders filteb by customer:
public function getOrderCollectionByCustomerId($customerId)
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('status',
['in' => $this->_orderConfig->getVisibleOnFrontStatuses()]
)
->setOrder(
'created_at',
'desc'
);
return $collection;
}
This method is helpful for creating customer dashboards or enhancing the “My Account” section.
Method 3: Get Order Collection Filter by Date
You can filter orders by a specific date range to identify trends or generate reports. Here is how we do it:
public function getOrderCollectionByDate($from, $to)
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('status',
['in' => $this->_orderConfig->getVisibleOnFrontStatuses()]
)
->setOrder(
'created_at',
'desc'
);
return $collection;
}
For example: Fetch orders placed within the last 7 days by passing $from = date(‘Y-m-d’, strtotime(‘-7 days’)) and $to = date(‘Y-m-d’).
Method 4: Get Order Collection Filter by Status
Filter orders based on their current status (e.g., pending, completed):
public function getOrderCollectionByStatus($statuses = [])
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('status',
['in' => $statuses]
)
;
return $collection;
}
Tip: Use Magento’s built-in status constants for better maintainability.
Method 5: Get Order Collection Filter by Payment Method
Besides the above solutions to help you get order collect filter, there is another way. The following guide will show you how you can get the order collection filter by payment method.
To filter orders by payment method, you’ll need to join the sales_order_payment table:
public function getOrderCollectionPaymentMethod($paymentMethod)
{
$collection = $this->_orderCollectionFactory()->create($customerId)
->addFieldToSelect('*')
->addFieldToFilter('created_at',
['gteq' => $from]
)
->addFieldToFilter('created_at',
['lteq' => $to]
);
/* join with payment table */
$collection->getSelect()
->join(
["sop" => "sales_order_payment"],
'main_table.entity_id = sop.parent_id',
array('method')
)
->where('sop.method = ?',$paymentMethod); //E.g: ccsave
$collection->setOrder(
'created_at',
'desc'
);
return $collection;
}
For example: Use ‘checkmo’ for Check/Money Order payment or ‘paypal_express’ for PayPal.
Best Practices for Getting Order Collections with Filters
- Optimize query performance: Design filters strategically to minimize the impact on performance, especially when working with large datasets. Use database indexes and efficient query structures to enhance speed and responsiveness.
- Thorough testing: Always test filter functionality in a development or staging environment before deploying to production. This ensures reliable performance and helps identify potential issues early.
- Ensure input security: Validate and sanitize all user inputs to protect against SQL injection and other vulnerabilities. Use parameterized queries or an ORM (Object-Relational Mapping) to handle input safely.
- Implement pagination: For large datasets, use pagination to improve performance and usability by reducing the load on the server and making data consumption easier for users.
- Document and standardize filters: Maintain clear documentation for the filters, including supported parameters and expected data types, to ensure consistency and ease of use across your development team.
Conclusion
Here are 5 simple methods to get all collection of orders which are filtered by different attributes such as customer, date, status, and payment method. I hope after reading this post, you will be able to apply the appropriate method in a specific situation in reality. If you have specific use cases or challenges, let us know in the comments—we’d love to help!
Related posts: