How to add Quick View feature in Magento 2
In some situations, you would want to preview the product right on the category page. In those cases, you can add the Quick view button on the product box on category listing. Whenever you clicked on it, a modal window that shows the product with all its functionality will be opened. However, the default setting does not allow you to do that. Therefore, in today’s post, I will guide you on how to add Quick View feature in Magento 2.
3 Steps to add Quick View feature:
- Step 1: Create and enable a Magento 2 module
- Step 2: Add some funcitionality
- Step 3: Edit JavaScript
Step 1: Create and enable a Magento 2 module
Firstly, you need to create app/code/Mageplaza/QuickView/registration.php
. Once it has been created, add the following code:
<?php
/**
* @category Mageplaza
* @package Mageplaza_QuickView
*/
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Mageplaza_QuickView',
__DIR__
);
The above code is very important to register your new module.
Besides, you need to create app/code/Mageplaza/QuickView/etc/module.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Mageplaza_QuickView" setup_version="1.0.0">
<sequence>
<module name="Magento_Catalog"/>
<module name="Magento_Customer"/>
</sequence>
</module>
</config>
and also app/code/Mageplaza/QuickView/composer.json
{
"name": "mageplaza/module-quickview",
"description": "Simple Quick View",
"require": {
"php": "~5.6.0|~7.0.0",
"magento/framework": "100.1.*"
},
"type": "magento2-module",
"version": "1.0.0",
"license": [
"proprietary"
],
"autoload": {
"files": [
"registration.php"
],
"psr-4": {
"Mageplaza\\QuickView\\": ""
}
}
}
Finally, you will be able to activate this extension when you run the following command:
php bin/magento module:enable Mageplaza_QuickView
Step 2: Add some funcitionality
To add quick view button on every products box in the category page, app/code/Mageplaza/QuickView/view/frontend/layout/catalog_category_view.xml
need to be created inside your project. And once you have created it, please add this:
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="content">
<referenceBlock name="category.product.addto">
<block class="Magento\Catalog\Block\Product\ProductList\Item\Block"
name="category.product.quickview"
as="quickview"
template="Mageplaza_QuickView::product/productlist/item/quickview.phtml"/>
</referenceBlock>
</referenceContainer>
</body>
</page>
The above code will allow you to add the button which is near the Add to cart button. You can style this button as your wish.
You should notice that you might probably need to remove several elements through XML so the product page in the modal window looks similar with the page on the product view page.
To do that, override Magento\Catalog\Controller\Product\View
and add new handle in case the product is called via iframe.
But before that, you need to create app/code/Mageplaza/QuickView/etc/frontend/di.xml
<?xml version="1.0"?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd">
<preference for="Magento\Catalog\Controller\Product\View"
type="Mageplaza\QuickView\Controller\Product\View" />
</config>
Then, you need app/code/Mageplaza/QuickView/Controller/Product/View.php
<?php
namespace Mageplaza\QuickView\Controller\Product;
use Magento\Catalog\Controller\Product\View as CatalogView;
/**
* Class View
*
* @package Mageplaza\QuickView\Controller\Product
*/
class View extends CatalogView
{
/**
* Overriden in order to add new layout handle in case product page is loaded in iframe
*
* @return \Magento\Framework\Controller\Result\Forward|\Magento\Framework\Controller\Result\Redirect
*/
public function execute()
{
if ($this->getRequest()->getParam("iframe")) {
$layout = $this->_view->getLayout();
$layout->getUpdate()->addHandle('quickview_product_view');
}
return parent::execute();
}
}
In other words, when product’s URL with iframe is called as GET parameter, its layout can be modify. Here is how you do it: app/code/Mageplaza/QuickView/view/frontend/layout/quickview_product_view.xml
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" layout="empty"
xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<update handle="empty"/>
<html>
<attribute name="class" value="quickview-scroll"/>
</html>
<body>
<attribute name="class" value="quickview-override"/>
<referenceContainer name="product.page.products.wrapper" remove="true" />
<referenceContainer name="product.info.details" remove="true" />
<referenceBlock name="reviews.tab" remove="true" />
<referenceBlock name="product.info.details" remove="true" />
<referenceBlock name="product.info.description" remove="true" />
<referenceBlock name="product.info.overview" remove="true" />
<referenceBlock name="authentication-popup" remove="true" />
</body>
</page>
The page layout can be edited as you want. For example, you can remove several elements.
Step 3: Edit JavaScript
This is the place where the magic will happen app/code/Mageplaza/QuickView/view/frontend/web/js/product/productlist/item/quickview.js
define([
'jquery',
'Magento_Ui/js/modal/modal',
'mage/loader',
'Magento_Customer/js/customer-data'
], function ($, modal, loader, customerData) {
'use strict';
return function(config, node) {
var product_id = jQuery(node).data('id');
var product_url = jQuery(node).data('url');
var options = {
type: 'popup',
responsive: true,
innerScroll: false,
title: $.mage.__('Quick View'),
buttons: [{
text: $.mage.__('Close'),
class: 'close-modal',
click: function () {
this.closeModal();
}
}]
};
var popup = modal(options, $('#quickViewContainer' + product_id));
$("#quickViewButton" + product_id).on("click", function () {
openQuickViewModal();
});
var openQuickViewModal = function () {
var modalContainer = $("#quickViewContainer" + product_id);
modalContainer.html(createIframe());
var iframe_selector = "#iFrame" + product_id;
$(iframe_selector).on("load", function () {
modalContainer.addClass("product-quickview");
modalContainer.modal('openModal');
this.style.height = this.contentWindow.document.body.scrollHeight+10 + 'px';
this.style.border = '0';
this.style.width = '100%';
observeAddToCart(this);
});
};
var observeAddToCart = function (iframe) {
var doc = iframe.contentWindow.document;
$(doc).contents().find('#product_addtocart_form').submit(function(e) {
e.preventDefault();
$.ajax({
data: $(this).serialize(),
type: $(this).attr('method'),
url: $(this).attr('action'),
success: function(response) {
customerData.reload("cart");
customerData.reload("messages");
$(".close-modal").trigger("click");
$('[data-block="minicart"]').find('[data-role="dropdownDialog"]').dropdownDialog("open");
}
});
});
};
var createIframe = function () {
return $('<iframe />', {
id: 'iFrame' + product_id,
src: product_url + "?iframe=1"
});
}
};
});
In here, you can find most of the bugs and fix it. It would be ideal if you use the default modal window of Magento, then open it using the button that you have just added in few steps earlier, create an iframe, load the product page in an iframe, and observe submit event on add to cart form. By doing that, when the product is added, you can close the window.
Conclusion
In conclusion, Quick View is a very useful tool, especially when you want to preview the product right on the category page. I hope that after reading this guide, you would have an idea about how to add Quick View feature in Magento 2.
Thanks for reading!
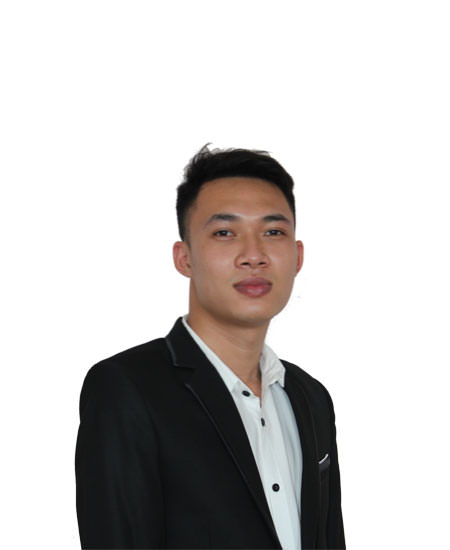
- How to create a simple Hello World module for Magento 2
- Magento 2 Block Template Ultimate Guides
- How to Create Module in Magento 2
- How to Create Controller in Magento 2
- How to create CRUD Models in Magento 2
- How to Create Magento 2 Block, Layout and Templates
- Configuration - System.xml
- How To Create Admin Menu In Magento 2
- Admin ACL
- Admin Grid
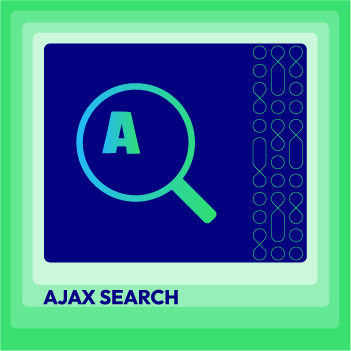
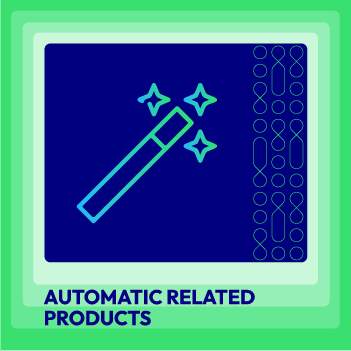
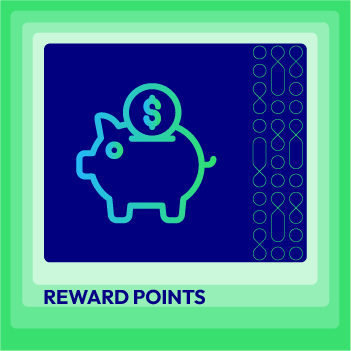
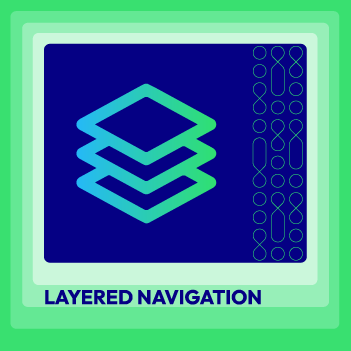
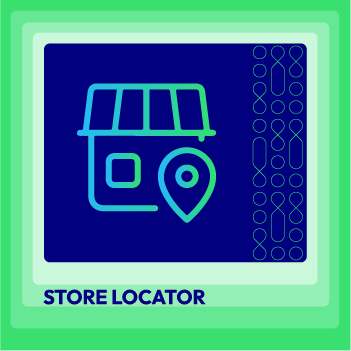