How to Create Magento 2 Block, Layout and Templates
In this topic, we will learn about View in Magento 2 including Block, Layouts and Templates. In previous topic, we discussed about CRUD Models. As you know, a View will be use to output representation of the page. In Magento 2, View is built by three path: block, layout and template. We will find how it work by building the simple module Hello World using View path.
To create view in Magento 2
- Step 1: Create controller
- Step 2: Create layout file .xml
- Step 3: Create block
- Step 4. Create template file .phtml
Step 1: Create controller
Firstly, We will create a controller to call the layout file .xml
File: app/code/Mageplaza/HelloWorld/Controller/Index/Display.php
<?php
namespace Mageplaza\HelloWorld\Controller\Index;
class Display extends \Magento\Framework\App\Action\Action
{
protected $_pageFactory;
public function __construct(
\Magento\Framework\App\Action\Context $context,
\Magento\Framework\View\Result\PageFactory $pageFactory)
{
$this->_pageFactory = $pageFactory;
return parent::__construct($context);
}
public function execute()
{
return $this->_pageFactory->create();
}
}
We have to declare the PageFactory and create it in execute method to render view.
Step 2: Create layout file .xml
The Layout is the major path of view layer in Magento 2 module. The layout file is a XML file which will define the page structure and will be locate in {module_root}/view/{area}/layout/
folder. The Area path can be frontend or adminhtml which define where the layout will be applied.
There is a special layout file name default.xml
which will be applied for all the page in it’s area. Otherwhile, the layout file will have name as format: {router_id}_{controller_name}_{action_name}.xml
.
You can understand the layout in detail in this Magento topic , and the instruction of a layout structure.
When rendering page, Magento will check the layout file to find the handle for the page and then load Block and Template. We will create a layout handle file for this module:
File: app/code/Mageplaza/HelloWorld/view/frontend/layout/helloworld_index_display.xml
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" layout="1column" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<referenceContainer name="content">
<block class="Mageplaza\HelloWorld\Block\Display" name="helloworld_display" template="Mageplaza_HelloWorld::sayhello.phtml" />
</referenceContainer>
</page>
In this file, we define the block and template for this page:
Block class: Mageplaza\HelloWorld\Block\Display
Template file: Mageplaza_HelloWorld::sayhello.phtml
name: It is the required attribute and is used to identify a block as a reference
Step 3: Create block
The Block file should contain all the view logic required, it should not contain any kind of html or css. Block file are supposed to have all application view logic.
Create a file:
app/code/Mageplaza/HelloWorld/Block/Display.php
Contents would be:
<?php
namespace Mageplaza\HelloWorld\Block;
class Display extends \Magento\Framework\View\Element\Template
{
public function __construct(\Magento\Framework\View\Element\Template\Context $context)
{
parent::__construct($context);
}
public function sayHello()
{
return __('Hello World');
}
}
Every block in Magento 2 must extend from Magento\Framework\View\Element\Template
. In this block we will define a method sayHello() to show the word “Hello World”. We will use it in template file.
Step 4. Create template file
Create a template file call sayhello.phtml
app/code/Mageplaza/HelloWorld/view/frontend/templates/sayhello.phtml
Insert the following code:
<?php
/**
* @var \Mageplaza\HelloWorld\Block\Display $block
*/
echo $block->sayHello();
In the layout file, we define the template by Mageplaza_HelloWorld::sayhello.phtml
. It mean that Magento will find the file name sayhello.phtml in templates folder of module Mageplaza_HelloWorld. The template folder of the module is app/code/{vendor_name}/{module_name}/view/frontend/templates/
.
In the template file, we can use the variable $block for the block object. As you see, we call the method sayHello()
in Block.
It’s done, please access to this page again (http://
In the previous Models (CRUD), we called postFactory model in controller. Now, we try show all data on table use block and template.
We edited file app/code/Mageplaza/HelloWorld/Block/Display.php
Contents would be:
<?php
namespace Mageplaza\HelloWorld\Block;
class Display extends \Magento\Framework\View\Element\Template
{
protected $_postFactory;
public function __construct(
\Magento\Framework\View\Element\Template\Context $context,
\Mageplaza\HelloWorld\Model\PostFactory $postFactory
)
{
$this->_postFactory = $postFactory;
parent::__construct($context);
}
public function sayHello()
{
return __('Hello World');
}
public function getPostCollection(){
$post = $this->_postFactory->create();
return $post->getCollection();
}
}
In block file we created a method getPostCollection
to get all data on mageplaza_helloworld_post
table and we will call it in template.
We edited file app/code/Mageplaza/HelloWorld/view/frontend/templates/sayhello.phtml
Contents would be:
<?php
/**
* @var \Mageplaza\HelloWorld\Block\Display $block
*/
echo $block->sayHello();
?>
<style>
table { font-family: arial, sans-serif; border-collapse: collapse; width: 100%; margin-top: 30px;}
td, th { border: 1px solid #dddddd; text-align: left; padding: 8px; }
tr:nth-child(even) { background-color: #dddddd; }
.post-id{width:2%} .post-name{width:30%}
</style>
<table>
<tr>
<th class="post-id">Id</th>
<th class="post-name">Name</th>
<th>Content</th>
</tr>
<?php
foreach ($block->getPostCollection() as $key=>$post){
echo '<tr>
<td>'.$post->getPostId().'</td>
<td>'.$post->getName().'</td>
<td>'.$post->getPostContent().'</td>
</tr>';
}
?>
</table>
</body>
</html>
After completing, please run php bin/magento cache:clean
and check the result. It will show like this
Exception printing is disabled by default for security reasons, this topic may help.
Related Post
FAQs
1. What is a block in Magento 2?
In Magento 2, a block is a PHP class that acts as an intermediary between the layout and the template. Blocks are responsible for providing the necessary data to the templates so they can render HTML. Essentially, blocks contain the logic needed to fetch data and pass it to the view layer (templates), which then displays this data on the front end of the website.
2. What is the difference between layout handles and layout XML files in Magento 2?
Layout handles are identifiers used within layout XML files to define specific configurations for different parts of the store (e.g., default, catalog_product_view). Layout XML files are the actual files that contain these configurations.
3. How do I add a custom template to a block in Magento 2?
In the block class, specify the template file using $this->setTemplate('Vendor_Module::path/to/template.phtml')
. Ensure the template file is placed in view/frontend/templates
.
4. How do I render a block in a Magento 2 template file?
To render a block, Use the getLayout()->createBlock('Vendor\Module\Block\ClassName')->setTemplate('Vendor_Module::path/to/template.phtml')->toHtml()
method within a template file.
5. How to override a core block or template in Magento 2?
To override a core block, create a preference in your module’s di.xml
. For templates, place your custom template in the appropriate path within your theme or module.
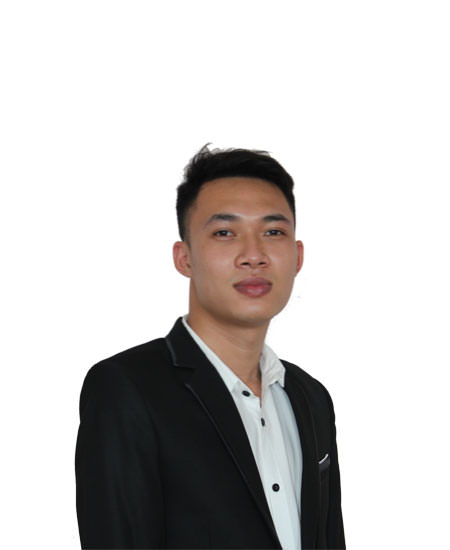
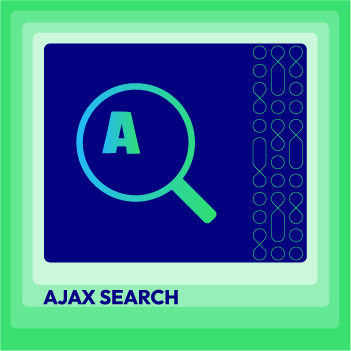
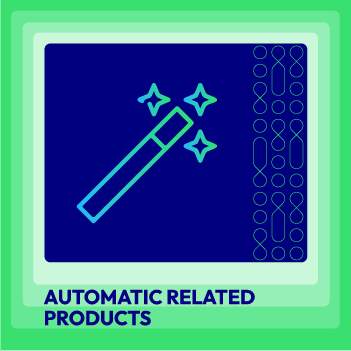
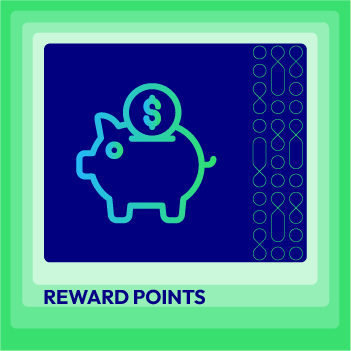
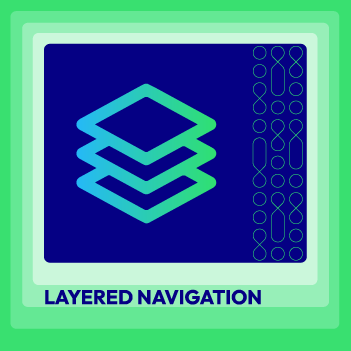
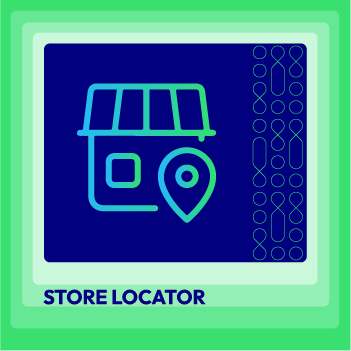
People also searched for
- magento 2 block
- magento 2 block class
- magento 2 block template
- magento 2 create a new template
- magento 2 create a template
- magento 2 create block
- magento 2 create block and template
- magento 2 create block from template
- magento 2 create block with template
- magento 2 create layout
- magento 2 create template
- magento 2 create template block
- magento 2 create template file
- magento 2 create template page
- magento 2 create template tutorial
- magento 2 custom template block
- magento 2 custom template cms
- magento 2 custom template for category
- magento 2 custom template for homepage
- magento 2 custom template for product
- magento 2 custom template layout
- magento 2 custom template not working
- magento 2 custom template tutorial
- magento 2 custom template variables
- magento 2 custom template xml
- magento 2 custom widget template
- magento 2 customer account create template
- magento 2 layout xml
- magento 2 create block programmatically
- magento 2 add block to layout
- magento 2 set template in block
- block in magento 2
- what is block in magento 2
- set template in block magento 2
- magento 2 product detail page template
- create the form in magento 2 front end with module
- 2.3.x, 2.4.x