Magento 2 Add Product Attribute Programmatically
In this article, we will find out how to create a product attribute in Magento 2 programatically. As you know, Magento 2 manage Product by EAV model, so we cannot simply add an attribute for product by adding a column for product table. Please read the article Magento 2 EAV model to understand about this.
Before we start with this, please read the article Magento 2 Install/Upgrade script to know how to create a module setup class.
Overview of Adding Product Attribute Programmatically
- Step 1: Create file InstallData.php
- Step 2: Define the install() method
- Step 3: Create custom attribute
In this article, we will use the Mageplaza HelloWorld module to learn how to add a product attribute.
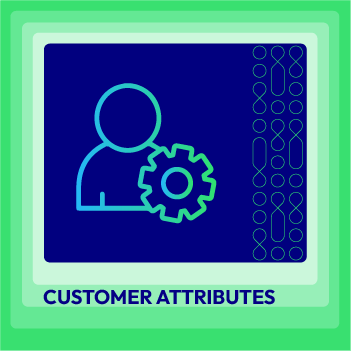
Customer Attributes for Magento 2
Enrich client data sources for better customer understanding and segmentation
Learn moreStep 1: Create file InstallData.php
We will start with the InstallData class which located in app/code/Mageplaza/HelloWorld/Setup/InstallData.php
. The content for this file:
<?php
namespace Mageplaza\HelloWorld\Setup;
use Magento\Eav\Setup\EavSetup;
use Magento\Eav\Setup\EavSetupFactory;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class InstallData implements InstallDataInterface
{
private $eavSetupFactory;
public function __construct(EavSetupFactory $eavSetupFactory)
{
$this->eavSetupFactory = $eavSetupFactory;
}
}
Step 2: Define the install() method
<?php
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
}
Step 3: Create custom attribute
Here are all lines code of InstallSchema.php to create product attribute programmically.
<?php
namespace Mageplaza\HelloWorld\Setup;
use Magento\Eav\Setup\EavSetup;
use Magento\Eav\Setup\EavSetupFactory;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class InstallData implements InstallDataInterface
{
private $eavSetupFactory;
public function __construct(EavSetupFactory $eavSetupFactory)
{
$this->eavSetupFactory = $eavSetupFactory;
}
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
$eavSetup->addAttribute(
\Magento\Catalog\Model\Product::ENTITY,
'sample_attribute',
[
'type' => 'text',
'backend' => '',
'frontend' => '',
'label' => 'Sample Atrribute',
'input' => 'text',
'class' => '',
'source' => '',
'global' => \Magento\Eav\Model\Entity\Attribute\ScopedAttributeInterface::SCOPE_GLOBAL,
'visible' => true,
'required' => true,
'user_defined' => false,
'default' => '',
'searchable' => false,
'filterable' => false,
'comparable' => false,
'visible_on_front' => false,
'used_in_product_listing' => true,
'unique' => false,
'apply_to' => ''
]
);
}
}
As you can see, all the addAttribute method requires is:
- The type id of the entity which we want to add attribute
- The name of the attribute
- An array of key value pairs to define the attribute such as group, input type, source, label…
All done, please run the upgrade script php bin/magento setup:upgrade
to install the module and the product attribute sample_attribute
will be created. After run upgrade
complete, please run php bin/magento setup:static-content:deploy
and go to product from admin to check the result. it will show like this:
If you want to remove product attribute, you can use method removeAttribute instead of addAttribute. It will be like this:
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
$eavSetup = $this->eavSetupFactory->create(['setup' => $setup]);
$eavSetup->removeAttribute(
\Magento\Catalog\Model\Product::ENTITY,
'sample_attribute');
}
Related Topics
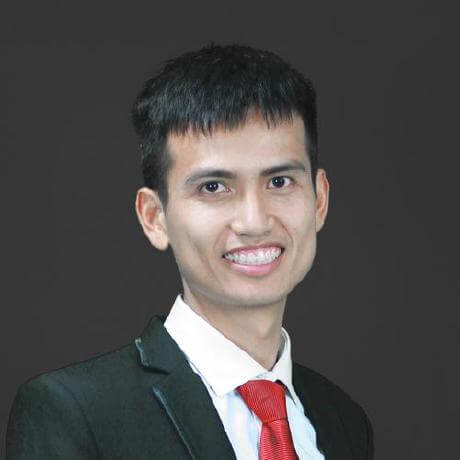
- How to create a simple Hello World module for Magento 2
- Magento 2 Block Template Ultimate Guides
- How to Create Module in Magento 2
- How to Create Controller in Magento 2
- How to create CRUD Models in Magento 2
- View: Layouts, Block and Templates
- Configuration - System.xml
- How To Create Admin Menu In Magento 2
- Admin ACL
- Admin Grid
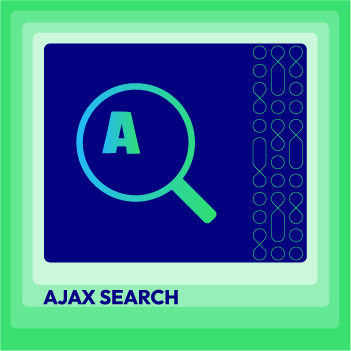
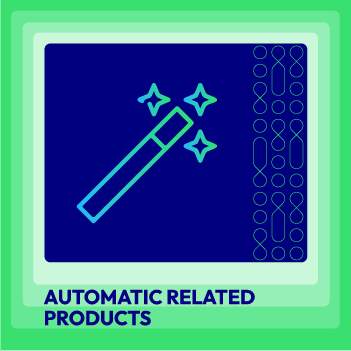
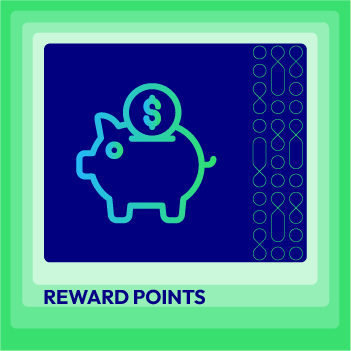
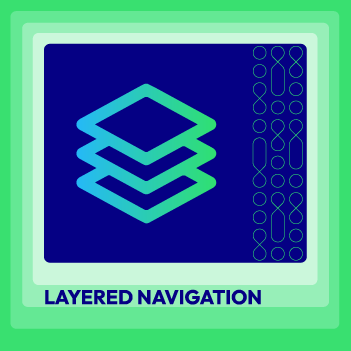
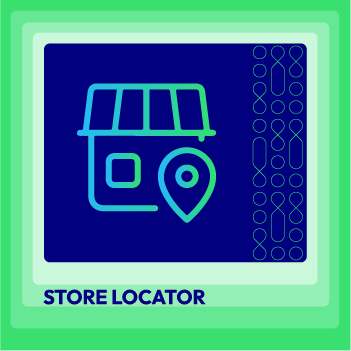
People also searched for
- Magento 2 Add Product Attribute Programmatically
- How to Add Eav Attribute for Product magento 2
- 2.3.x, 2.4.x