How to Get Categories from Specific Product in Magento 2?
Vinh Jacker | 03-17-2025
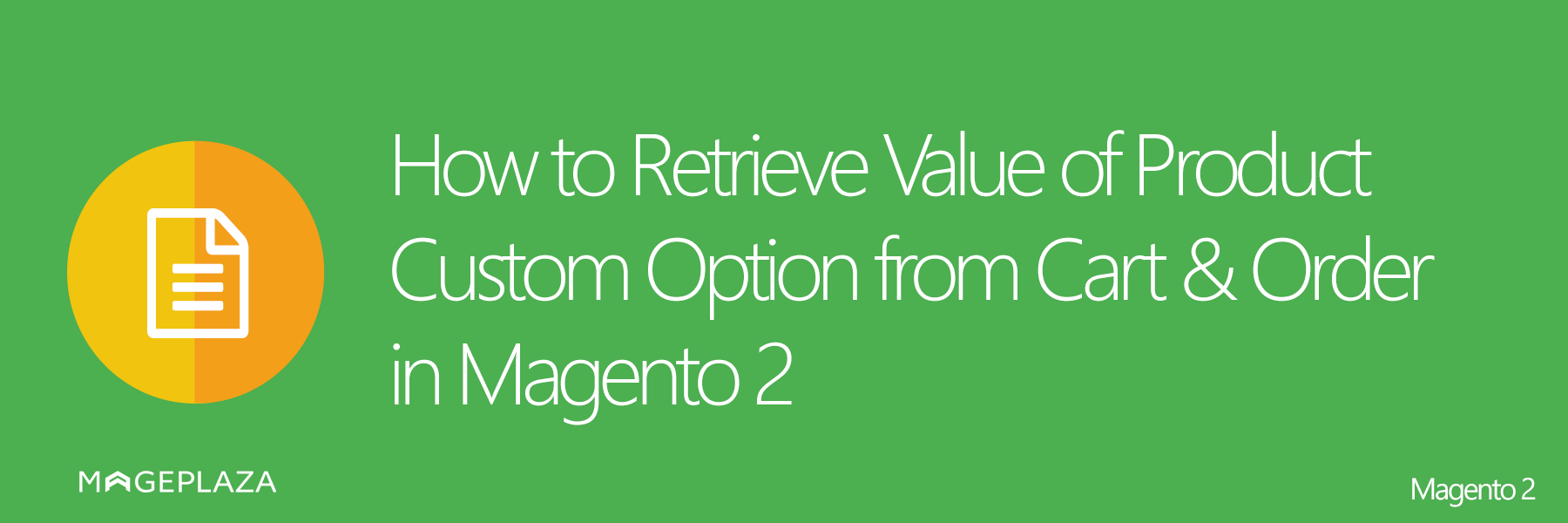
Sometimes you might need to collect the entire product list or product category from a specific product you are seeing. A quick solution can be used to acquire a product category without going back and forth within your website. You’ll get the categories from any product you want in a time-saving and accurate manner.
Now let’s see how to get categories from specific products in Magento 2. This method enables you to get the list from current or any product as you need.
At first, you need to open the block class of my custom module (Mageplaza_HelloWorld
), then inject the object of \Magento\Catalog\Model\ResourceModel\Category\CollectionFactory
, \Magento\Catalog\Model\ProductRepository
and \Magento\Framework\Registry
classes in the constructor of that block class.
app/code/Mageplaza/HelloWorld/Block/HelloWorld.php
<?php
namespace Mageplaza\HelloWorld\Block;
class HelloWorld extends \Magento\Framework\View\Element\Template
{
protected $_categoryCollectionFactory;
protected $_productRepository;
protected $_registry;
public function __construct(
\Magento\Backend\Block\Template\Context $context,
\Magento\Catalog\Model\ResourceModel\Category\CollectionFactory $categoryCollectionFactory,
\Magento\Catalog\Model\ProductRepository $productRepository,
\Magento\Framework\Registry $registry,
array $data = []
)
{
$this->_categoryCollectionFactory = $categoryCollectionFactory;
$this->_productRepository = $productRepository;
$this->_registry = $registry;
parent::__construct($context, $data);
}
/**
* Get category collection
*
* @param bool $isActive
* @param bool|int $level
* @param bool|string $sortBy
* @param bool|int $pageSize
* @return \Magento\Catalog\Model\ResourceModel\Category\Collection or array
*/
public function getCategoryCollection($isActive = true, $level = false, $sortBy = false, $pageSize = false)
{
$collection = $this->_categoryCollectionFactory->create();
$collection->addAttributeToSelect('*');
// select only active categories
if ($isActive) {
$collection->addIsActiveFilter();
}
// select categories of certain level
if ($level) {
$collection->addLevelFilter($level);
}
// sort categories by some value
if ($sortBy) {
$collection->addOrderField($sortBy);
}
// select certain number of categories
if ($pageSize) {
$collection->setPageSize($pageSize);
}
return $collection;
}
public function getProductById($id)
{
return $this->_productRepository->getById($id);
}
public function getCurrentProduct()
{
return $this->_registry->registry('current_product');
}
}
?>
In case you want to work with the current product, the getCurrentProduct()
funciton is active. And if from any specific item, please enable getProductById($id)
function. After that, you can retrieve the collection of the category from the category IDs which are linked to that product. In the .phtml
file, you need to add the following code snippet:
$productId = 1; // YOUR PRODUCT ID
$product = $block->getProductById($productId);
// for current product
// $product = $block->getCurrentProduct();
$categoryIds = $product->getCategoryIds();
$categories = $block->getCategoryCollection()
->addAttributeToFilter('entity_id', $categoryIds);
foreach ($categories as $category) {
echo $category->getName() . '<br>';
}
Conclusion
Those are all things you will use to retrieve categories from specific products in Magento 2. Following the above steps makes it easier and quicker for you to get things done. It saves time and doesn’t affect any existing product setup on your website. Thanks for your reading and please comment below if there is any trouble with that.
Recommend Topics