How to add custom validations before order placement Magento 2
This article will help you add your own validation before placing an order.
This is the step where you add a new field and want to verify that field. For example, in the checkout, if a customer hasn’t selected input for a required field, the validation event will be triggered before he or she clicks on Place Order. This feature is helpful because it notifies customers of the missing information before completing the form.
Since we don’t add any new field, we will verify an existing field, the email field.
We will only allow orders with customers’ email from Google (Gmail) to go through.
Add custom validations before processing orders.
- Step 1: Create the validator.
- Step 2: Add validator to the validators pool.
- Step 3: Declare the validation in the checkout layout.
- Step 4: Test the new validator.
You should know how to create a basic Magento 2 module. All of customized files will be inside this module.
Let’s dive in the steps to add custom validations before order placement.
Step 1: Create the validator.
Create isGmail.js
in Mageplaza/HelloWorld/view/frontend/web/js/model
directory.
validate
method is required
define(
[
'jquery',
'mage/validation'
],
function ($) {
'use strict';
return {
/**
* Validate checkout agreements
*
* @returns {Boolean}
*/
validate: function () {
var emailValidationResult = false;
var email = $('form[data-role=email-with-possible-login]').val();
if(~email.search("gmail.com")){ //should use Regular expression in real store.
emailValidationResult = true;
}
return emailValidationResult;
}
};
}
);
Step 2: Add validator to the validators pool.
Create isGmail.js
in Mageplaza/HelloWorld/view/frontend/web/js/view
directory.
define(
[
'uiComponent',
'Magento_Checkout/js/model/payment/additional-validators',
'Mageplaza_HelloWorld/js/model/isGmail'
],
function (Component, additionalValidators, gmailValidation) {
'use strict';
additionalValidators.registerValidator(gmailValidation);
return Component.extend({});
}
);
Step 3: Declare the validation in the checkout layout.
Add the following code to Mageplaza/HelloWorld/view/frontend/layout/checkout_index_index.xml
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" layout="1column" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceBlock name="checkout.root">
<arguments>
<argument name="jsLayout" xsi:type="array">
<item name="components" xsi:type="array">
<item name="checkout" xsi:type="array">
<item name="children" xsi:type="array">
<item name="steps" xsi:type="array">
<item name="children" xsi:type="array">
<item name="billing-step" xsi:type="array">
<item name="children" xsi:type="array">
<item name="payment" xsi:type="array">
<item name="children" xsi:type="array">
<item name="additional-payment-validators" xsi:type="array">
<item name="children" xsi:type="array">
<!-- Declare your validation. START -->
<item name="gmailValidation" xsi:type="array">
<item name="component" xsi:type="string">Mageplaza_HelloWorld/js/view/isGmail</item>
</item>
<!-- Declare your validation. END -->
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</item>
</argument>
</arguments>
</referenceBlock>
</body>
</page>
Step 4: Test the new validator.
Now time to flush cache and try to checkout with different emails. If you have any issue, feel free to leave a comment below, Mageplaza and Magento community are willing to help.
Wrap up
That’s all about adding custom validations before order placement in Magento 2. I hope you find the right tutorial for your work. If you have any questions, feel free to let me know. Thanks for reading!
- How to create a simple Hello World module for Magento 2
- Magento 2 Block Template Ultimate Guides
- How to Create Module in Magento 2
- How to Create Controller in Magento 2
- How to create CRUD Models in Magento 2
- How to Create Magento 2 Block, Layout and Templates
- Configuration - System.xml
- How To Create Admin Menu In Magento 2
- Admin ACL
- Admin Grid
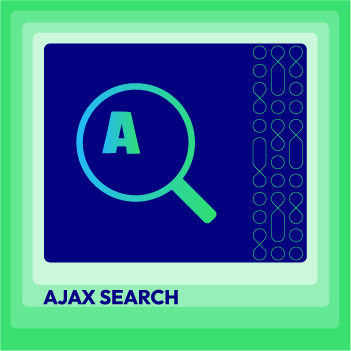
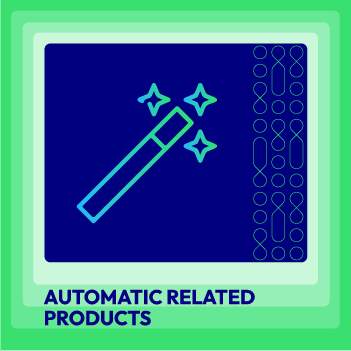
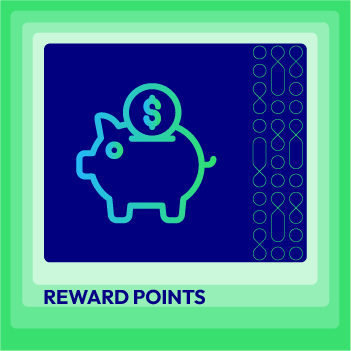
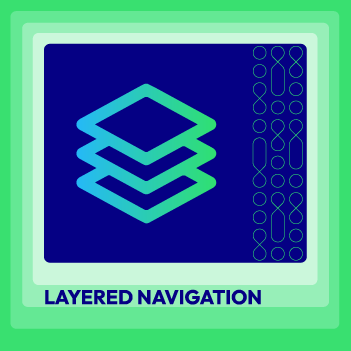
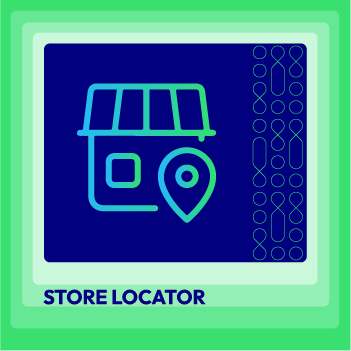
People also searched for
- magento 2 add custom validation checkout
- magento 2 checkout custom field validation
- magento 2 checkout validation
- magento 2 checkout phone number validation
- magento 2 checkout field validation
- magento 2 checkout form validation
- custom detail validation customer
- magento 2 add custom validation
- magento 2 order verification
- magento 2 override validation js
- 2.3.x, 2.4.x