How to Add Items in Shopware
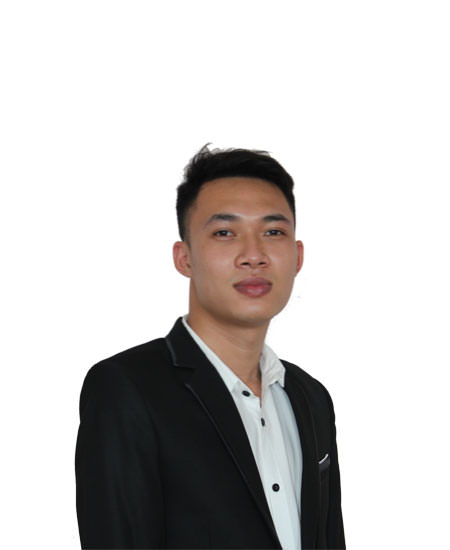
With a big catalog of 224+ extensions for your online store
As you are an owner of your Shopware business, you need to know how to add items in Shopware in order to take your online career to the next level. By knowing this, you can keep your products and items under better control. Then, you can be good at working with items in your Shopware.
Adding items is not complicated to perform. However, it will require some coding skills to make the task accomplished. Moreover, you will also need to how to customize them to make them match your Shopware store. What all we could help you complete this job. Now, follow us with some simple steps and you will be a professor in adding items.
An overview of Shopware items
In your Shopware, items will function as one of the principal entities. When it comes to knowing what it actually is, it will mostly be determined by the real platform solution. In general, items refer to the term that can match what you will have in the cart perfectly; however, it could be regarded as very generic.
How to add items in Shopware
Now it is time to learn how to add items in Shopware. There are only two simple steps. The first one is to add one simple item and the second one is to construct a brand-new factory handler. In this tutorial, you can make use of the Storefront controller example.
Step 1: Add one simple item
In order to add the simple item, you can make use of the example to be already registered. Nevertheless, it is not necessary to have a controller here. It only provides the benefit of fetching your contemporary cart by using \Shopware\Core\Checkout\Cart\Cart. This can be added as one method argument that could be filled automatically by the argument resolver.
In case, you are going to use it for any other thing, not a controller, it is possible for you to fetch the contemporary cart with a method
\Shopware\Core\Checkout\Cart\SalesChannel\CartService::getCart
. So, do not hesitate to add one product example to the cart that uses code. In this situation, you are supposed to access the service \Shopware\Core\Checkout\Cart\LineItemFactoryRegistry as well as the \Shopware\Core\Checkout\Cart\SalesChannel\CartService
that is supplied to the service or controller through Dependency injection.
In order to help you understand better, you could look at one typical example as below:
<?php declare(strict_types=1);
namespace Swag\BasicExample\Service;
use Shopware\Core\Checkout\Cart\LineItem\LineItem;
use Shopware\Core\Checkout\Cart\LineItemFactoryRegistry;
use Shopware\Core\Checkout\Cart\SalesChannel\CartService;
use Shopware\Core\Framework\Routing\Annotation\RouteScope;
use Shopware\Core\System\SalesChannel\SalesChannelContext;
use Shopware\Storefront\Controller\StorefrontController;
use Shopware\Storefront\Framework\Routing\StorefrontResponse;
use Symfony\Component\Routing\Annotation\Route;
use Shopware\Core\Checkout\Cart\Cart;
/**
* @RouteScope(scopes={"storefront"})
*/
class ExampleController extends StorefrontController
{
private LineItemFactoryRegistry $factory;
private CartService $cartService;
public function __construct(LineItemFactoryRegistry $factory, CartService $cartService)
{
$this->factory = $factory;
$this->cartService = $cartService;
}
/**
* @Route("/cartAdd", name="frontend.example", methods={"GET"})
*/
public function add(Cart $cart, SalesChannelContext $context): StorefrontResponse
{
// Create product line item
$lineItem = $this->factory->create([
'type' => LineItem::PRODUCT_LINE_ITEM_TYPE, // Results in 'product'
'referencedId' => 'myExampleId', // this is not a valid UUID, change this to your actual ID!
'quantity' => 5,
'payload' => ['key' => 'value']
], $context);
$this->cartService->add($cart, $lineItem, $context);
return $this->renderStorefront('@Storefront/storefront/base.html.twig');
}
}
As we have previously mentioned, it is possible for you to apply the argument to your methods, then it can be filled automatically. After that, you could be able to construct one line item with the use of LineItemFactoryRegistry, coupled with its created method. What is more, it is also necessary that you should supply the property type. And it could be one item among the below ones by default: product, promotion, credit, and custom.
To be more specific, you could notice that the LineItemFactoryRegistry will hold a load of handlers for creating the line item for a certain type. Each type of line item requires one handler that will be later indicated in this tutorial. In case, this type isn’t supported, then it tends to throw an exception \Shopware\Core\Checkout\Cart\Exception\LineItemTypeNotSupportedException.
Moreover, applying the referenced is also what you could do. In case you are keen on adding one line item that belongs to the promotion type, then the “referencedId” aims at pointing to the corresponding promotion ID. More than that, the field quantity only includes the line-item quantity, which you will add to your cart.
Now, it is time for you to have a glance at the field payload that only comprises the dummy data. This field payload tends to include any extra needed data in order to be attached to one line item for handling the business logic properly. For example, the information related to the selected option of one configurable product will be saved there.
So, it is possible for you to apply this for applying vital information to the line item in which you need to perform later on. Moreover, in case you call this route /cartAdd, it will add the product and item with the myExampleIdID to your cart.
Step 2: Construct a brand-new factory handler
Move on to the next step with constructing a brand-new factory handler. In several cases, you are fond of having a customized handler of the line item for your new entity, including one bundle entity. In this situation, it is possible for you to construct your own new handler that can be available within the LineItemFactoryRegistry and this can be considered the valid option type.
Furthermore, you are required to construct one new class that tends to implement the interface \Shopware\Core\Checkout\Cart\LineItemFactoryHandler\LineItemFactoryInterface.
Likewise, it is necessary that it is registered in its DI container that comes with its tag “shopware.cart.line_item.factory”.
<?xml version="1.0" ?>
<container xmlns="http://symfony.com/schema/dic/services"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://symfony.com/schema/dic/services http://symfony.com/schema/dic/services/services-1.0.xsd">
<services>
<service id="Swag\BasicExample\Service\ExampleHandler">
<tag name="shopware.cart.line_item.factory" />
</service>
</services>
</container>
Check out the below example handler:
<?php declare(strict_types=1);
namespace Swag\BasicExample\Service;
use Shopware\Core\Checkout\Cart\LineItem\LineItem;
use Shopware\Core\Checkout\Cart\LineItemFactoryHandler\LineItemFactoryInterface;
use Shopware\Core\System\SalesChannel\SalesChannelContext;
class ExampleHandler implements LineItemFactoryInterface
{
public const TYPE = 'example';
public function supports(string $type): bool
{
return $type === self::TYPE;
}
public function create(array $data, SalesChannelContext $context): LineItem
{
return new LineItem($data['id'], self::TYPE, $data['referencedId'] ?? null, 1);
}
public function update(LineItem $lineItem, array $data, SalesChannelContext $context): void
{
if (isset($data['referencedId'])) {
$lineItem->setReferencedId($data['referencedId']);
}
}
}
By executing the LineItemFactoryInterface, it forces you to execute another three methods.
-
Supports: This method will be applied the $type string. In this case, the handler will support the type of line-item example.
-
Create: This kind of method will be accounted for constructing the instance of the LineItem. So, you can apply all vital things for the customized type of line item, including fields.
-
Update: This kind of method will be called the update of your LineItemFactoryRegistry. As the name suggests, the line item can be updated. In this situation, you are capable of defining what type of properties that belong to the line item can be updated. To be more particular, in case you prefer that property X contains Y, you could perform so here.
Now, you have to add the processor for this type, or else the item will not be persisted within your cart. One simple processor will look like below.
<?php declare(strict_types=1);
namespace Swag\BasicExample\Service;
use Shopware\Core\Checkout\Cart\LineItem\LineItem;
use Shopware\Core\Checkout\Cart\LineItemFactoryHandler\LineItemFactoryInterface;
use Shopware\Core\System\SalesChannel\SalesChannelContext;
class ExampleHandler implements LineItemFactoryInterface
{
public const TYPE = 'example';
public function supports(string $type): bool
{
return $type === self::TYPE;
}
public function create(array $data, SalesChannelContext $context): LineItem
{
return new LineItem($data['id'], self::TYPE, $data['referencedId'] ?? null, 1);
}
public function update(LineItem $lineItem, array $data, SalesChannelContext $context): void
{
if (isset($data['referencedId'])) {
$lineItem->setReferencedId($data['referencedId']);
}
}
}
As you could notice, this kind of processor will take the original cart as one input for adding every instance of the example types to the second cart. This will be persisted.
And you could also make use of processors for doing more than that. Now, it is time for you to register this kind of processor within the services.xml:
...
<services>
...
<service id="Swag\BasicExample\Core\Checkout\Cart\ExampleProcessor">
<tag name="shopware.cart.processor" priority="4800"/>
</service>
</services>
Conclusion
This tutorial is perfect for those who are looking for how to add items to Shopware. With some kinds of coding, you can make your online store more professional and look attractive in the eyes of customers and visitors to your site. Now, go with us on this guide and you can accomplish the task effortlessly. Hope that you will be interested in this article and keep following us for more great tips in the upcoming time.
Increase sales,
not your workload
Simple, powerful tools to grow your business. Easy to use, quick to master and all at an affordable price.
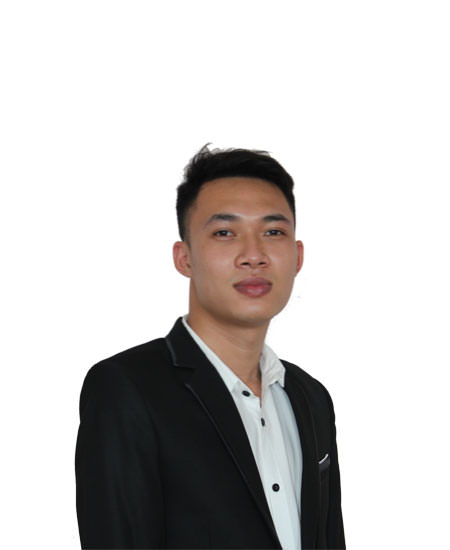
Recent Tutorials
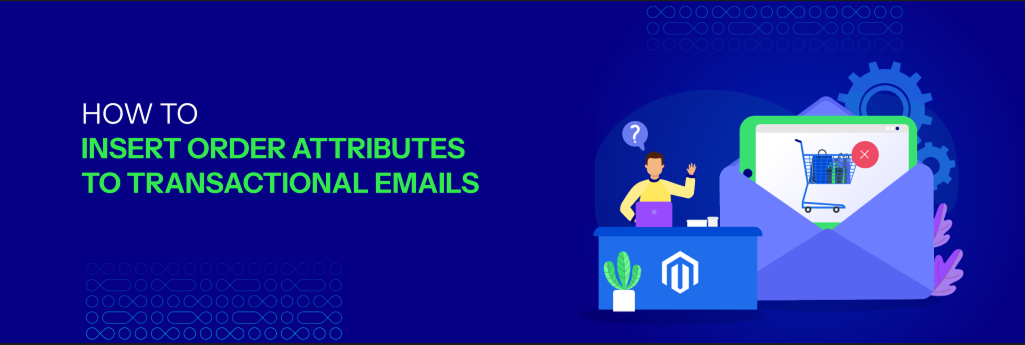
How to insert Order Attributes to Transactional Emails - Mageplaza
How to add Order Attributes to PDF Order Template - Mageplaza
Setup Facebook Product Feed for Magento 2 - Mageplaza
Explore Our Products:
Stay in the know
Get special offers on the latest news from Mageplaza.
Earn $10 in reward now!