JavaScript New Features: ECMAScript® 2024 (ES15) Updates
Javascript, the biggest workhorse behind the breath-taking and inspiring webpages keeps on evolving and moving up its speed every single day. The existence of the largest user base of 63.61% of developers, who are using it in their projects with 1st place in the ranking of the most used programming languages developers around the world in 2023 (Statista), makes it a ruler in the programming languages world.
These 2024 updates mean an optimistic projection of the future of JavaScript if you are a JavaScript fanatic or a web developer who wants to stay a step ahead of the competition. Therefore, apply the seatbelt and find out how these new features will amplify your JavaScript coding skills.
Hire JavaScript Developers
ECMAScript® 2024 (ES15) Release
Every year, JavaScript comes with an upgrade to the official specification of ECMAScript, which defines the language. And would you believe it, the next release, ECMAScript® 2024 (ES15), is just full of goodies that are worth the hype!
These new language features aren’t just about fancy syntax. They address real-world developer pain points and bring powerful tools to our coding arsenals. By understanding and using them, you’ll write cleaner, more maintainable, and future-proof JavaScript.
It’s important to understand that features go through various stages before becoming fully baked into a release. Here’s a quick breakdown:
- Stage 0 (Strawperson): A new idea surfaces. It’s still a very early stage.
- Stage 1 (Proposal): Formal definition of the feature, but still malleable.
- Stage 2 (Draft): Syntax and semantics are getting nailed down.
- Stage 3 (Candidate): The feature is nearly finalized, and broader feedback is sought.
- Stage 4 (Finished): It’s officially part of the language
The ES15 release includes features promoted to Stage 4 and some exciting ones still in the pipeline that might just make their way into future releases.
So, what’s in store? Let’s explore a few of the major highlights that deserve your attention in the ES15 release. We’ll cover features focused on readability, new paradigms, and much more in the rest of this blog!
JavaScript New Features
The ECMAScript 2024 update brings a suite of exciting new features to JavaScript. Let’s dive in and explore how these additions can enhance your coding experience.
Enhancing Code Readability and Efficiency
In the world of programming, readable and efficient code is the holy grail. It makes your code easier to understand (for both you and your future collaborators!), less prone to errors, and can even boost performance. The 2024 JavaScript updates directly target these goals with some fantastic additions.
- No More Function Wrappings: Top-level await liberates you from this pattern! Now you can use await directly at the top level of your modules. This seemingly small change streamlines asynchronous code, especially when dealing with module-level operations.
Before | After |
---|---|
|
|
The Pipe Operator (|): The pipe operator introduces a more intuitive way of chaining functions, making your code flow easier to read. Think of it as a visual representation of data being passed through a series of transformations. The pipe operator leads to less nested code and a clearer representation of the sequence of operations.
Before | After |
---|---|
|
|
- Top-level await and the pipe operator are not just about fancy syntax; they promote clean, easily understandable code.
- These features are especially helpful when dealing with asynchronous operations and multiple data transformations.
Introducing New Paradigms
Records and Tuples
Records and tuples introduce the concepts of true immutability to JavaScript. What does this mean?
- Records: Think of them as objects with values that cannot be changed after creation.
- Tuples: Ordered, fixed-length lists where the elements cannot be modified.
Why immutability matters:
- Predictability: Immutability prevents accidental changes to data, reducing the potential for unexpected bugs.
- Concurrency: Immutable data structures are safer to work with in multi-threaded environments (like web workers).
- Functional Programming: Records and tuples align well with functional programming principles, leading to more elegant and maintainable code.
Pattern Matching
Pattern matching offers a powerful and expressive way to handle conditional logic. It allows you to compare your data against specific patterns and execute code accordingly. Think of it like an advanced ‘switch’ statement with superpowers! See this example for more:
const result = match (value) {
when { status: 'success', data: _ } => // Handle successful response
when { status: 'error', code: 404 } => // Handle 404 error
when _ => // Default case
}
Records and tuples encourage a data-centric approach focused on immutability. Pattern matching enables cleaner and more expressive ways to write conditional logic. These new paradigms open doors to writing JavaScript code in styles that were less practical before.
Taming Time with the Temporal API
If you’ve ever had the misfortune of dealing with time and date maneuvering in JavaScript, you’ve seen many people in the same situation before! The old Date library is a constant headache to many developers as it has so many peculiarities and restrictions. The Temporal API is aimed at letting you live a more hassle-free and easy life.
Why the Temporal API?
- Intuitive: The Temporal API introduces new classes like `PlainDate`, `PlainTime`, `Instant`, and `ZonedDateTime`, representing specific date and time concepts without the ambiguity of the old `Date` object.
- Timezone Aware: The Temporal API handles timezones gracefully, allowing you to perform calculations and conversions with confidence. No more confusing timezone offsets!
- Immutable: Temporal objects are immutable by default, helping prevent accidental modifications that lead to subtle bugs.
- Built for the Modern World: The Temporal API supports non-Gregorian calendars and advanced formatting demanded by internationalization.
Let’s see it in action, this is an example for getting the current date and time:
const now = Temporal.Now.plainDateTimeISO();
console.log(now.toString());
Adding/subtracting time:
const yesterday = now.subtract({ days: 1 });
const nextWeek = now.add({ weeks: 1 });
Timezone conversions:
const timezone = Temporal.TimeZone.from('America/Los_Angeles');
const laTime = now.withTimeZone(timezone);
The Temporal API comes as a one-stop solution to what has been bothering JS programmers since time immemorial – managing dates and times properly. Mastering the Temporal API means less time spent battling date quirks and more time focused on building amazing features.
Temporal API is a big leap that makes JavaScript language beyond the doubt for dealing with time-related data. Give yourself time to navigate through its functions and you will discover that it can be indeed a very helpful tool.
Unique Point: Exploring Decorators
Decorators are an exciting experimental feature that could potentially change the way we write and organize JavaScript code. While not yet officially part of the ECMAScript 2024 release, they’ve got quite a buzz around them, so let’s take a closer look!
Think of decorators as functions that can enhance the functionality of other functions, classes, or even class properties. They use a special syntax, starting with the @ symbol, placed directly before the target they modify.
Why should you care?
- Code Readability: Decorators will enable you to implement logic to existing code without making it messy with supplementary conditions.
- Separation of Concerns: Modules maintain code cleanliness and the cross-cutting concerns can be separated into reusable decorators to do logging, authentication, of validation.
- Flexibility: Decorators are dynamic—applied at runtime, giving you more control over how they modify behavior.
Let’s imagine a @log decorator:
function log(target, name, descriptor) {
const originalMethod = descriptor.value;
descriptor.value = function(...args) {
console.log(`Calling method: ${name}`);
const result = originalMethod.apply(this, args);
console.log(`Result: ${result}`);
return result;
};
}
class MyClass {
@log
myMethod(arg) {
return arg * 2;
}
}
Decorators are still at an early stage, but they hold the potential to streamline common coding patterns. They encourage a more declarative style, where you focus on what to achieve, not the intricate details of how.
Additional Features to Watch Out For
The JavaScript world is in a constant state of evolution, and the 2024 update brings even more goodies in the pipeline. Let’s take a quick glance at some other noteworthy additions and proposals that deserve to be on your radar:
- Object.groupBy: This handy method makes it easy to group elements of an array based on a specified property. Imagine streamlining data organization in just one line of code!
- Array.prototype.toReversed/toSorted/toSpliced: Tired of workaround solutions for non-mutating array manipulation? These new methods allow you to create reversed, sorted, or spliced copies of your arrays, keeping the originals intact.
- Array.prototype.findLast/findLastIndex: Finding the last element (or its index) that matches a condition within an array becomes a breeze with these dedicated methods.
- JSON Import Assertions: A way to ensure data integrity and security by specifying the expected content type of a JSON module directly during import.
- Pipeline Operator variations: Proposals exist for variations of the pipe operator, such as the ‘|>` (F# style) and ‘^^’ (elixir style), offering more stylistic choices in composing functions.
These features showcase the ongoing efforts to make JavaScript even more expressive and developer-friendly. While some may still be in the experimental stage, they provide a glimpse into the potential future directions of the language.
Need Expert JavaScript Assistance?
It’s no secret that JavaScript is the driving force behind modern web interactions. To achieve truly dynamic, engaging, and performant web experiences, partnering with skilled JavaScript developers is often a necessity.
Mageplaza’s team of meticulously vetted JavaScript specialists is here to propel your projects forward. We offer expertise in
- Crafting dynamic user interfaces: Building responsive, interactive elements that keep visitors engaged.
- Performance Optimization: Ensuring your web applications load quickly and deliver a seamless user experience.
- Feature Implementation: Harnessing the latest JavaScript features to enhance your website’s functionality and add that “wow” factor.
- Troubleshooting Experts: Resolving complex JavaScript issues and ensuring your codebase remains robust.
Start that web development journey of yours now and take it to a higher level with us by checking out how our amazing JavaScript skills can be useful to your projects.
CONTACT OUR JAVASCRIPT EXPERTS TODAY
Conclusion
The JavaScript 2024 update manifests a torrent of innovations that allude to the role of the language as the foundation of modern web development. From cleaner code with top-level await and the pipe operator to the paradigm shifts introduced by records and tuples, and the powerful Temporal API, JavaScript is becoming more expressive than ever. And with exciting features like decorators on the horizon, the future looks even brighter.
Stay curious, keep learning, and embrace these new tools to take your development skills to the next level!
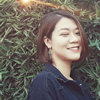
& Maintenance Services
Make sure your store is not only in good shape but also thriving with a professional team yet at an affordable price.
Get StartedNew Posts
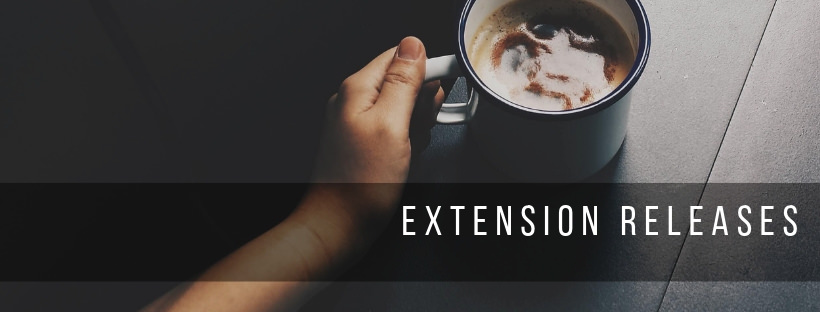
May 2024
People also searched for
- JavaScript New Features
- 2.3.x, 2.4.x
Stay in the know
Get special offers on the latest news from Mageplaza.
Earn $10 in reward now!